Servlet:a short introduction:-
A servlet handles the requests from the server, that require servlet to add something or do some operations in response to the client request so that a dynamic page can be generated, rather than the same old monotonous static html pages.
The servlet API's are generally provided along with Containers like tomcat, JBoss etc and you have to import the servlet-api.jar for compiling your servlet .java files. A java servlet does not have a main method so it is the container that controls the execution of servlet methods. The container initializes the servlet by calling it's init method, after which it's service method is called which determines that which of the servlet's method is to be called depending upon the client request and after a servlet's job is done it purges it's resources by calling it's destroy method.
The container also provides support for JSP.
The servlet class that you will implement let it's name be MyServlet , will generally extend the HttpServlet class that extends the GenericServlet class so the class hierarchy will be as shown in the following figure.
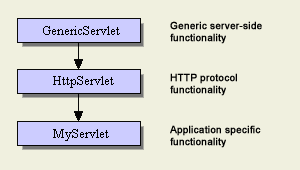
The servlet class that you will implement will most likely implement either of the following two methods:-
- public void doGet(HttpServletRequest request,HttpServletResponse response) throws IOException{ //code here}:-
If the client uses the http Get query, then this will be the method that will be invoked by the container to handle the Get request. It takes reference to two objects HttpServletRequest and HttpServletResponse object, these objects are created by the container and their reference is passed to this method. A client will use a Get http request, If the amount of data to be sent is less, the data sent using get request is visible in the browser navigation bar, so you would not want to display sensitive information like user password, for that you will want to use the Http Post request . - public void doPost(HttpServletRequest request,HttpServletResponse response) throws IOException{ // code here }
If the client uses the http post request then this will be the method called by the container by looking at the client request. It is used when the amount of data to be sent is more, like filling up the html form and sending it to the server to process it and also when sensitive information like user password is to sent.
The example servlet(infoServlet) will be the most basic servlet, It will take the parameter user-name and password, perform some fake authentication (authentication won't actually be done) and display a welcome message to the user along with the current date and time.
The servlet code is given below and the explanation follows:-
import javax.servlet.*; import javax.servlet.http.*; import java.io.*; public class infoServlet extends HttpServlet{ public void doPost(HttpServletRequest request, HttpServletResponse response)throws IOException,ServletException {response.setContentType("text/html"); PrintWriter output=response.getWriter(); String name=request.getParameter("text_field"); String password=request.getParameter("password_field"); Date now = new Date(); //No authentication will be done output.println("<html>"+"<head>"+"<title>"+ "Welcome page"+"</title>"+"</head>"+ "<body>"+"Welcome "+name+"<br/>"+ "The time is"+now+"</body>"+"</html>"); }
In this servlet we have implemented the doPost method as the client will be sending the sensitive information in a post request.
The method setContentType("content_type"); tells about the response mime type to the client browser so that it can display the page received properly.
Then we obtain a printwriter object reference by invoking the getWriter() method on the response object, which is used to generate the dynamic response page for the client.
The getParameter("field_name") gets the parameter value entered by the user in the form.
Now save the java file as infoServlet.java .
Compiling the servlet:-
To compile the servlet follow these steps:-
- Open the command prompt and navigate to the directory where the infoServlet java file is saved.
- Now to compile the servlet execute the following command.
javac -classpath "C:/Program files/Tomcat6.0/lib/servlet_api.jar" infoServlet.java
Here replace the classpath (the path between the quotes) with the path to your servlet_api.jar file provided by the container that you are using.
I am here using the tomcat container, the deploying part may vary depending upon the container that you use.
- Create a directory under the tomcat6.0/webapps directory, name it proj1 then under the proj1 directory create a directory WEB-INF , then create a directory classes under the WEB-INF directory.
- Copy the generated class file into the classes directory.
Now you would have to create the deployment descriptor ie the web.xml file that tells the container about information such as where the servlet class file is placed, what is the deployment name that you are using, what will be the name by which client will call the servlet and obviously various other parameters.
The deployment descriptor is given in the following code-box, copy the contents and save it as web.xml
<?xml version="1.0"?> <web-app xmlns="http://java.sun.com/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" version="2.5"> <servlet> <servlet-name>TestServlet</servlet-name> <servlet-class>infoServlet</servlet-class> </servlet> <servlet-mapping> <servlet-name>TestServlet</servlet-name> <url-pattern>/submit</url-pattern> </servlet-mapping> </web-app>
Explanation:-
One should not worry about the webapp tag just copy and paste it.
The <servlet> tag:- It defines the properties relating to your servlet. In this case we map the deployment name ie the name specified by <servlet-name> tag , to the path to actual class file i.e the name specified by the <servlet-class> tag; i.e the infoservlet, notice here that we do not specify the name as infoServlet.java as it is the java class file.
The <servlet-mapping > tag :-It is used to map the name by which the client will access the servlet to the deployment name. Here the <url-pattern> specifies the name by which the client accesses the servlet.
- Deploying (Contd .....) : Copy the web.xml file and save it under the WEB-INF directory.
- Now we would have to create the simple html form in which user will enter the details and when he clicks on the submit button the post request will be send to the server. The container will then invoke the servlet to do its operation and servlet will generate the response which will be converted to http repsonse by the container and send back to the client. Simple isn't it. The code for the form is given below, copy and paste the code and save it as form.html under the proj1 directory.
<html> <head> <title> A temporary page </title> </head> <body> <h1> Fill up the following form </h1> <form id="2314" method="post" action="submit"> <center> Enter your name <input name="text_field" type="text" value=""/><br/><br/> Enter your password <input name="password_field" type="password" value="" /> <br/><br/> <br/> <input type="submit" value="submit form"> </center> </form> </body> </html>
The html code is pretty simple and you should note only 2 things :-- The form is using the post method so a post query is generated when the user clicks on the submit button.
- The input field name property is used by the servlet to access the parameter values entered by the user.
Phew! everything is done now just the working of application is to be seen.
- Start the apache tomcat service. Note in case you have problems launching it just go to its binary directory i.e the bin directory and launch it from there, it will be launched in a command prompt.
- Open the browser and access the html form and type http://localhost:8080/proj1/form.html in your browser navigation bar.
- Fill up the details and click on the submit button and voila! you will see the response generated by the servlet which will be the user-name and the date that you entered in the form.